原文:
Introduction
Suppose you have been asked to migrate an existing multi-tier application to .NET where the business layer is written in Java. Normally you would have no option but to recode and port the entire application to any .NET language (e.g. C#). However this is where IKVM.NET comes to the rescue.
IKVM.NET is an open source implementation of Java for Mono /Microsoft .NET Framework and makes it possible both to develop .NET applications in Java, and to use existing Java API's and libraries in applications written in any .NET language. It is written in C# and the executables, documentation and source code can be downloaded from here.
IKVM.NET consists of the following three main parts:
- A Java Virtual Machine implemented in .NET
- A .NET implementation of the Java class libraries
- Tools that enable Java and .NET interoperability
However before we get any further into this topic, let’s discuss about few of the main components of the IKVM.NET package which we would be using later in this article.
-
IKVM.Runtime.dll: The VM runtime and all supporting code containing the byte code JIT compiler/verifier, object model remapping infrastructure and the managed .NET re-implementations of the native methods in Classpath.
-
IKVM.GNU.Classpath.dll: Compiled version of GNU Classpath, the Free Software Foundation's implementation of the Java class libraries, plus some additional IKVM.NET specific code.
-
ikvm.exe: Starter executable, comparable to java.exe ("dynamic mode").
-
ikvmc.exe: Static compiler. Used to compile Java classes and jars into a .NET assembly ("static mode").
Now back to our problem of migrating the existing Java business classes so that they can be accessed by the newly proposed .NET application. We would also like to use the various existing Java API and libraries in our .NET application. Let’s start by doing just that.
Setting Up IKVM.NET
Download the binary distribution from the sourceforge site and unzip the contents to C:\ikvm (or X:\ikvm where X is your drive). You would find the ikvm executables and DLLs in the C:\ikvm\bin directory. Open a command or shell window, cd to C:\ikvm\bin, and type ‘ikvm’.
If your system is operating correctly, you should see the following output:
usage: ikvm [-options] <class> [args...] (to execute a class) or ikvm -jar [-options] <jarfile> [args...] (to execute a jar file)
For Linux based systems, the setup is similar as above. This is all you need to do for running the demo application.
Our Demo Java Business Class (JavaToNet.java)
Collapse
Copy Code
public class JavaToNet
{
public static void main(String[] args)
{
System.out.println("This is a demonstration Program which\n");
System.out.println("shows the conversion of Java class to\n");
System.out.println("a .NET dll\n");
}
public static double AddNumbers(double a,double b){
double c = 0;
c = a + b;
return c;
}
public static double SubNumbers(double a,double b){
double c = 0;
c = a - b;
return c;
}
public static double MulNumbers(double a,double b){
double c = 0;
c = a * b;
return c;
}
public static double DivNumbers(double a,double b){
double c = 0;
c = a / b;
return c;
}
}
Our Java class is very simple. It has four functions for add, subtract, multiply and divide that take two double values and return a result. Our objective is to access these functions through our C# application. Compile the above Java file to get the JavaToNet.class. We will use this Java class file to generate the .NET DLL to be referenced in our C# program.
Using IKVM.NET to Convert Java Class to .NET DLL
Copy the above Java class file (JavaToNet.class) to the C:\ikvm\bin directory. Now run the following command:

This would create the JavaToNet.dll from the JavaToNet.class file. There are other command line option for ikvmc.exe. For example: ‘ikvmc –target:exe javaToNet.class
’ would create an EXE and not a DLL. You can get all the options by typing ‘ikvmc’ in the command line.
Setting Up Your .NET Development Environment
-
Start by creating a C# Windows application project.
-
Drag and drop controls into the form as shown:
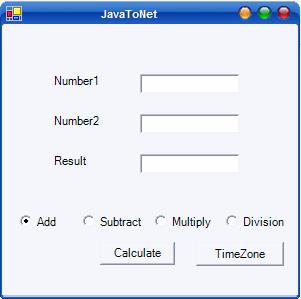
-
Add the following DLLs as references to the project. Both DLLs are present in the C:\ikvm\bin folder.
-
JavaToNet.dll
-
IKVM.GNU.Classpath.dll
-
Add the following code to the button click event of the ‘Calculate’ button:
Collapse
Copy Code
private void btnCal_Click(object sender, System.EventArgs e)
{
if (rdAdd.Checked == true)
{
txtResult.Text = Convert.ToString(JavaToNet.AddNumbers
(Convert.ToDouble(txtNum1.Text),Convert.ToDouble(txtNum2.Text)));
}else if (rdSub.Checked ==true)
{
txtResult.Text = Convert.ToString(JavaToNet.SubNumbers
(Convert.ToDouble(txtNum1.Text),Convert.ToDouble(txtNum2.Text)));
}
else if (rdMul.Checked == true)
{
txtResult.Text = Convert.ToString(JavaToNet.MulNumbers
(Convert.ToDouble(txtNum1.Text),Convert.ToDouble(txtNum2.Text)));
}
else
{
txtResult.Text = Convert.ToString(JavaToNet.DivNumbers
(Convert.ToDouble(txtNum1.Text),Convert.ToDouble(txtNum2.Text)));
}
}
-
Add the following using
directive on the top of the *.cs file:
Collapse
Copy Code
using TimeZone = java.util.TimeZone;
-
Add the following code to the button click event of the ‘Time Zone’ button.
Collapse
Copy Code
private void btnTimeZone_Click(object sender, System.EventArgs e)
{
MessageBox.Show(TimeZone.getDefault().getDisplayName());
}
-
Compile and run the application. The C# application would now call the AddNumbers()
, SubNumbers()
, MulNumbers()
and DivNumbers()
functions present in the JavaToNet.dll and return the result.
-
Click on the ‘Time Zone’ button. The application accesses the java.util.TimeZone
class and displays the exact time zone of the place.
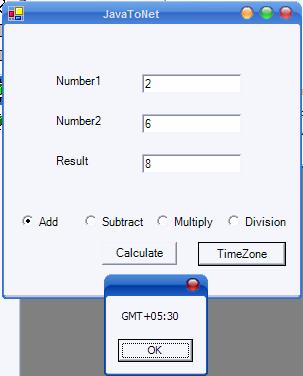
Conclusion
Since these methods had originally been written in Java, IKVM.NET provides us an easy and viable way to access those classes and methods from a .NET application. Similarly as shown above in the ‘Time Zone’ example, you can access most of the existing Java packages (e.g. java.io, java.util, etc.) and use them in your application.
However there are certain drawbacks. IKVM.NET, while still being actively developed, has limited support for AWT classes and hence porting Java GUI can be ruled out at present. Also some of the default Java classes are still being ported so you might not get all the functionalities you require. Also if your application depends on the exact Java class loading semantics, you might have to modify it to suit your needs.
=============================================================================
译著:
在.net程序中使用java类
假设你曾经这样被要求:把分层开发项目中由java编写的业务逻辑层迁移到.net平台上。
通常情况下你是没选择的,必须重写编写代码并且将实体层转换成.NET平台的任意一种语言(例如 C#).
遇到这种情况是不是无计与施了呢?
问题不是没有办法解决的,IKVM.NET便因此而生。
IKVM.NET是一个用C#编写的开源项目,它是Mono/Microsoft.NET Framework项目java版的实现,
它实现了用java开发.NET程序的可能,同时还可以将程序中现有的java API和程序集
用任意一种.NET语言实现.IKVM.NET程序的源代码和参考文档可以在这里下载.
IKVM.NET主要由以下三部分组成:
1.用.NET实现的Java虚拟机
2 用.NET实现的java类库
3.实现了Java与.NET之间交互的一些工具类
在我们深入探讨这个专题之前,不妨让我们来了解一下文章中将要用到的IKVM.NET包中的
一些重要的组件.
•IKVM.Runtime.dll: 虚拟机(VM)运行时、含有字节码(byte code)即时(JIT)编译/校验器的
所有代码集;对象模型的底层映射;
Classpath路径中程序集的、本地化方法的、托管.NET资源的重实现.
•IKVM.GNU.Classpath.dll:已编译的GNU版本的Classpath,由the Free Software Foundation's
(免费软件基金会)添加了一些 IKVM.NET特有用途代码的Java类库
•ikvm.exe: 启动程序, 相比于java.exe ("动态模式").
•ikvmc.exe: 编译器. 将Java的类和jars编译成.NET程序集 ("静态模式").
好了,让我们回到将Java业务逻辑层迁移到.NET平台,用于其他用途的问题上来。
我们通常也会在我们的.NET程序中大量使用现有Java API的可能。开始我们的有趣之旅吧。
搭建起IKVM.NET
从sourceforge下载回程序后解压到 c:\ikvm(或者 x:\ikvm, x是你电脑上驱动盘符的路径).
解压后接可以在c:\ikvm\bind的文件夹下找到ikvm可执行程序和dll文件.
打开命令提示符或shell窗口,切换到 c:\ikvm\bin目录,输入'ikvm'.
如果你的操作系统正确的安装了,你将会看到以下内容:
usage: ikvm [-options] <class> [args...] (to execute a class) or ikvm -jar [-options] <jarfile> [args...] (to execute a jar file)
基于Linux的操作系统安装方法与上面的方法相似.
好了,运行演示程序前的工作已经做好了.
public class JavaToNet
{
public static void main(String[] args)
{
System.out.println("This is a demonstration Program which\n");
System.out.println("shows the conversion of Java class to\n");
System.out.println("a .NET dll\n");
}
public static double AddNumbers(double a,double b){
double c = 0;
c = a + b;
return c;
}
public static double SubNumbers(double a,double b){
double c = 0;
c = a - b;
return c;
}
public static double MulNumbers(double a,double b){
double c = 0;
c = a * b;
return c;
}
public static double DivNumbers(double a,double b){
double c = 0;
c = a / b;
return c;
}
}
我们的java类非常见,它有四个函数,分别是加、减、乘、除,两个
double行操作数和一个返回值.我们的目标是通过C#程序访问这些函数.
编译以上java文件,生成JavaToNet.class文件。我们将会用java类文件
生成.net的dll文件,供我们的程序引用.
用IKVM.NET将java类文件转换成.NET的dll文件
将以上java类文件(JavaToNet.class)复制到C:\ikvm\bin目录下.
并运行下面的命令ikvm -target:library JavaToNet.class
以上操作将会通过JavaToNet.class生成一个JavaToNet.dll
ikvmc还有一些其他命令行参数,例如:'ikvmc -target:exe JavaToNet.class'
将会创建一个exe文件而并非dll文件.你可以输入'ikvm'获得所有命令行帮助.
架设起你的.NET开发环境
1.创建一个 C# windows项目
2.按照以下界面进行拖拽控件
3.将以下程序添加到项目的引用中,所有dll文件都可以在c:\ikvm\bin文件夹中找到
◦JavaToNet.dll
◦IKVM.GNU.Classpath.dll
4.将 以下代码添加到按钮Calculate的点击事件中
private void btnCal_Click(object sender, System.EventArgs e)
{
if (rdAdd.Checked == true)
{
txtResult.Text = Convert.ToString(JavaToNet.AddNumbers
(Convert.ToDouble(txtNum1.Text),Convert.ToDouble(txtNum2.Text)));
}else if (rdSub.Checked ==true)
{
txtResult.Text = Convert.ToString(JavaToNet.SubNumbers
(Convert.ToDouble(txtNum1.Text),Convert.ToDouble(txtNum2.Text)));
}
else if (rdMul.Checked == true)
{
txtResult.Text = Convert.ToString(JavaToNet.MulNumbers
(Convert.ToDouble(txtNum1.Text),Convert.ToDouble(txtNum2.Text)));
}
else
{
txtResult.Text = Convert.ToString(JavaToNet.DivNumbers
(Convert.ToDouble(txtNum1.Text),Convert.ToDouble(txtNum2.Text)));
}
}
5. 在*.cs的文件头处添加以下引用
using TimeZone = java.util.TimeZone;
6 将 以下代码添加到按钮Time Zone的点击事件中
private void btnTimeZone_Click(object sender, System.EventArgs e)
{
MessageBox.Show(TimeZone.getDefault().getDisplayName());
}
7.编译并运行程序. C#现在将会调用JavaToNet.dll中的AddNumbers(),
SubNumbers(), MulNumbers() 和 DivNumbers()函数,并且返回结果.
8.点击'Time Zone'按钮,程序将会访问java.util.TimeZone类,并且显示
所处位置的时区.
总结:
既然这些方法起初已经是用java编写,IKVM在.NET程序中却为我们提供一个
便捷的、多途径的方法访问这些类的方法.就上面那个'Time Zone'来说,你可以
访问Java包(例如 java.io,java.util, 等)并且在你的程序中使用它。
当然程序肯定是存在内部联系的。IKVM.NET 仍然积极的在发展着,他对AWT类的
支持却受到限制,因而Java GUI这一块目前就被划去了.尽管java中的某些默认类
未编制到程序中,所以你可能找不到你所需的函数。如果你的程序依赖于java类
加载的特性,你就需要按照你所需进行适当调整了.
笔者思: 公司中,项目大一点动辄就100多张表,要是单单把项目从java向.net
平台迁移,重写实体层非累死人,如果有公司的实体生成器倒要好一点,
生成后稍稍改改代码就OK,但你有没有想过不动实体层就实现项目从一个
平台向另一个平台的迁移呢.... 这要省多少事,还有那些无意中冒出的未知的
头疼的事情呢.
按照作者的原意来说,java项目向.net迁移时,只要不涉及AWT相关可视化控件的
类都可以无缝迁移到.NET平台. 有了IKVM.NET后 '我(程序源代码)'在java这边也是
对象操作,到.NET那边还是对象操作.变平台,但是类之间的通讯方法仍然和娘家(Java)那边
一模一样. 呵呵
累了 没时间整理,把作者文章的大意拿过来,没怎么整理,只要大家看懂便是:) 不做过多介绍
自己去悟吧 嘿嘿~~
原文参考地址 http://www.codeproject.com/KB/dotnet/csharpikvm.aspx
分享到:
相关推荐
AOP in .NET introduces aspect-oriented programming to .NET developers and provides practical guidance on how to get the most benefit from this technique in your everyday coding. The book's many ...
在ASP.NET中使用WINDOWS验证方式连接SQL SERVER数据库 改进ADO.Net数据库访问方式 ASP.NET 2.0 绑定高级技巧 简单实用的DataSet更新数据库的类+总结 [ADO.NET]由数据库触发器引发的问题 为ASP.NET封装的SQL数据库...
If you are interested in the product, you can contact us by e-mail to the address Java4Less@confluencia.net. http://rreport.port5.com http://rreport.8m.com http://www.java4less.com EVALUATION ...
your program to use IMAP instead of POP and expect everything in IMAP to be supported. Assuming your mail server supports IMAP, your JavaMail-based program can take Fundamentals of the JavaMail API...
So, if you want to generate or work with PDF files as part of your ASP.NET web application, you will have to rely on one of the many third party components that are available. Google will help you to...
Covers debugging, errors, comparisons with C++ and Java, classes and arrays, variables, and more Includes access to a companion website with sample code and bonus materials Everything you need to make...
的 Android SDK 提供了在 Android 平台上使用 JaVa 语言进行 Android 应用开发必须的工具和 API 接口。 特性 • 应用程序框架 支持组件的重用与替换 • Dalvik Dalvik Dalvik Dalvik 虚拟机 专为移动设备优化 • ...
In fact, the power of C# and the easy-to-learn syntax persuaded many developers to switch to it from C++ or Java. The C# 2005 version (also referred to as C# 2.0) added even more powerful features to...
Your Application Does Something, Right? Achieving the Minimum Version=Control Emulators and Targets Virtually There Aiming at a Target ■Chapter 3: Creating a Skeleton Application Begin at the ...
All open test architecture API classes and methods can be referenced from the VAPI-XP user interface so that you can easily include them in your test script. About Working with System Tests You ...
Choosing to use IBM MQ classes for Java or IBM MQ classes for JMS.............................................. 45 Design techniques for messages..........................................................